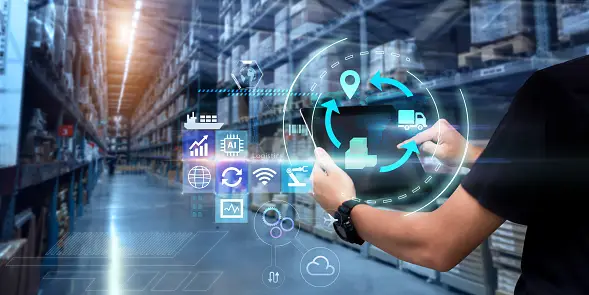
Step-by-Step: Building a DIY IoT Project for Beginners In 2024? The Internet of Things has become an integral part of modern technology, allowing everyday objects to communicate and share data over the internet. Our project will provide you with hands-on experience in working with microcontrollers, sensors, and cloud platforms, setting the foundation for your exploration into the world of IoT.
Whether you’re a hobbyist, a student, or someone curious about IoT, this guide aims to make the learning process enjoyable and accessible. So, let’s embark on this journey together and build your own IoT project from scratch!
Table of Contents
ToggleDefine Your Project
Decide on the purpose of your IoT project. In this case, we’ll monitor temperature and humidity.
Choose Components
Select the necessary components for your project:
- Microcontroller (e.g., Arduino, Raspberry Pi)
- Temperature and humidity sensor (e.g., DHT11, DHT22)
- WiFi module (if not built into the microcontroller)
- Breadboard and jumper wires
- Power source (battery or USB power)
Set Up the Microcontroller
Connect the temperature and humidity sensor to the microcontroller following its datasheet.
If you’re using Arduino, install the Arduino IDE on your computer.
Write a simple program to read data from the sensor.
#include <DHT.h>
#define DHTPIN 2 // Pin where the sensor is connected
#define DHTTYPE DHT22 // DHT 22 (AM2302) sensor type
DHT dht(DHTPIN, DHTTYPE);
void setup() {
Serial.begin(9600);
dht.begin();
}
void loop() {
delay(2000); // Wait for 2 seconds between measurements
float humidity = dht.readHumidity();
float temperature = dht.readTemperature();
Serial.print("Humidity: ");
Serial.print(humidity);
Serial.print("%\t");
Serial.print("Temperature: ");
Serial.print(temperature);
Serial.println("°C");
}
Connect to the Internet
If your microcontroller doesn’t have built-in WiFi, connect a WiFi module (e.g., ESP8266) to enable internet connectivity.
Modify your code to include WiFi credentials and send data to a cloud platform.
#include <DHT.h>
#include <ESP8266WiFi.h>
#include <WiFiClient.h>
#define DHTPIN 2
#define DHTTYPE DHT22
const char *ssid = "YourSSID";
const char *password = "YourPassword";
const char *server = "api.yourcloudplatform.com";
const int port = 80;
DHT dht(DHTPIN, DHTTYPE);
void setup() {
Serial.begin(9600);
dht.begin();
WiFi.begin(ssid, password);
while (WiFi.status() != WL_CONNECTED) {
delay(1000);
Serial.println("Connecting to WiFi...");
}
}
void loop() {
delay(2000);
float humidity = dht.readHumidity();
float temperature = dht.readTemperature();
Serial.print("Humidity: ");
Serial.print(humidity);
Serial.print("%\t");
Serial.print("Temperature: ");
Serial.print(temperature);
Serial.println("°C");
// Send data to the cloud
WiFiClient client;
if (client.connect(server, port)) {
String data = "humidity=" + String(humidity) + "&temperature=" + String(temperature);
client.print("POST /update_data HTTP/1.1\n");
client.print("Host: " + String(server) + "\n");
client.print("Content-Length: " + String(data.length()) + "\n");
client.print("Content-Type: application/x-www-form-urlencoded\n\n");
client.print(data);
delay(1000); // Wait for response
client.stop();
}
}
Create Cloud Platform Account
Sign up for an account on a cloud platform (e.g., ThingSpeak, Blynk, or your custom server). Obtain the necessary API keys or credentials.
Update Code for Cloud Integration
Modify the code to use the API keys or credentials from your cloud platform. For example, if using ThingSpeak, replace the server
variable and update the data
string accordingly.
Monitor Data
Upload the modified code to your microcontroller. Open the serial monitor in the Arduino IDE to check if the data is being read and sent successfully.
Step 8: View Data on Cloud Platform
Visit your cloud platform’s dashboard to view the real-time data from your IoT device.
Congratulations! You’ve built a simple IoT project for monitoring temperature and humidity. This project can be expanded by adding more sensors, incorporating actuators, or integrating with other IoT devices.
Read More; 3 Factors That Made Blockchain Become Valuable Last Year
Visualize Data with a Dashboard
Many cloud platforms offer customizable dashboards. Explore your platform’s dashboard settings and create visualizations for the temperature and humidity data.
Add graphs, gauges, or charts to make the data more visually appealing and easier to understand.
Implement Notifications
Enhance your IoT project by adding notification features. For example, you can receive an email or push notification when the temperature or humidity crosses a certain threshold.
Modify your code to include conditional statements that trigger notifications.
Power Management
Depending on your power source, implement power-saving measures to extend the device’s battery life.
Consider adding a sleep mode between data readings and wake up the device at predefined intervals.
Secure Your IoT Device
If applicable, implement security measures to protect your IoT device and data. For instance, use HTTPS for communication with the cloud platform.
Change default login credentials, and if possible, encrypt the data being transmitted.
Mobile App Integration
Develop a simple mobile app to monitor the IoT device remotely.
Platforms like Blynk or MIT App Inventor can help you create basic apps without extensive coding.
Expand Sensor Array
Add more sensors (e.g., light, motion, gas) to collect diverse data.
Modify your code to accommodate the new sensors and send the data to the cloud.
Data Analytics
Explore data analytics tools to derive insights from the collected data.
Consider integrating machine learning algorithms for predictive analysis.
Firmware Over-the-Air (FOTA) Updates
Implement FOTA updates to remotely update your device’s firmware.
This allows you to fix bugs or add features without physically accessing the device.
Community and Documentation
Share your project with the IoT community.
Document your project thoroughly, including code comments, a README file, and a circuit diagram.
Troubleshooting and Maintenance
Develop a plan for troubleshooting common issues.
Regularly update your device’s software and firmware to ensure optimal performance.
Cost Optimization
Optimize your project for cost by exploring more affordable components or alternative cloud platforms.
Consider power-efficient components to reduce energy consumption.
Future Improvements
Continuously brainstorm and implement improvements to your IoT project.
Stay updated with new technologies and trends in the IoT space for inspiration.
Conclusion
Building a DIY IoT project can be a rewarding experience, providing hands-on learning and practical insights into the world of connected devices. Throughout this step-by-step guide, you’ve learned how to create a basic temperature and humidity monitoring system, and then expanded it to include features like cloud integration, visualization, notifications, and more.
FAQs : DIY IoT Project
Q1: Can I use a different microcontroller for this project?
A1: Yes, you can choose a microcontroller based on your preferences and project requirements. Popular choices include Arduino, Raspberry Pi, ESP8266, or ESP32.
Q2: How do I choose the right cloud platform for my IoT project?
A2: Consider factors such as ease of use, cost, scalability, and available features. Platforms like ThingSpeak, Blynk, and AWS IoT are commonly used and have extensive documentation.
Q3: Can I add more sensors to the project?
A3: Absolutely! You can expand the sensor array by adding more sensors based on your interests or application needs. Ensure your microcontroller has sufficient pins and processing power.
Q4: What if I want to add actuators to control devices based on sensor data?
A4: You can extend the project by incorporating actuators (like relays or servos) into the circuit and modifying the code to control devices based on sensor data.
Q5: Is it possible to integrate voice control into the IoT project
? A5: Yes, you can integrate voice control using platforms like Amazon Alexa, Google Assistant, or custom voice recognition systems.
Q6: How can I secure my IoT device from potential threats?
A6: Implement security measures such as using encrypted communication protocols (HTTPS), changing default credentials, and keeping your device’s firmware updated.